chrome.bookmarks
Description: |
Use the chrome.bookmarks API to create, organize, and otherwise manipulate bookmarks. Also see Override Pages, which you can use to create a custom Bookmark Manager page.
|
Availability: |
Since Chrome 35.
|
Permissions: |
"bookmarks"
|
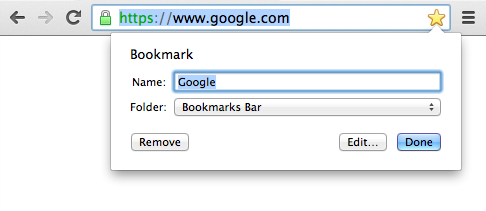
Manifest
You must declare the "bookmarks" permission in the extension manifest to use the bookmarks API. For example:
{ "name": "My extension", ... "permissions": [ "bookmarks" ], ... }
Objects and properties
Bookmarks are organized in a tree, where each node in the tree is either a bookmark or a folder (sometimes called a group). Each node in the tree is represented by a bookmarks.BookmarkTreeNode object.
BookmarkTreeNode
properties
are used throughout the chrome.bookmarks
API.
For example, when you call
bookmarks.create,
you pass in the new node's parent (parentId
),
and, optionally, the node's
index
, title
, and url
properties.
See bookmarks.BookmarkTreeNode
for information about the properties a node can have.
Note: You cannot use this API to add or remove entries in the root folder. You also cannot rename, move, or remove the special "Bookmarks Bar" and "Other Bookmarks" folders.
Examples
The following code creates a folder with the title "Extension bookmarks".
The first argument to create()
specifies properties
for the new folder.
The second argument defines a function
to be executed after the folder is created.
chrome.bookmarks.create({'parentId': bookmarkBar.id, 'title': 'Extension bookmarks'}, function(newFolder) { console.log("added folder: " + newFolder.title); });
The next snippet creates a bookmark pointing to the developer documentation for extensions. Since nothing bad will happen if creating the bookmark fails, this code doesn't bother to define a callback function.
chrome.bookmarks.create({'parentId': extensionsFolderId, 'title': 'Extensions doc', 'url': 'http://code.google.com/chrome/extensions'});
For an example of using this API, see the basic bookmarks sample. For other examples and for help in viewing the source code, see Samples.
Summary
Types | |
---|---|
BookmarkTreeNodeUnmodifiable | |
BookmarkTreeNode | |
Properties | |
MAX_WRITE_OPERATIONS_PER_HOUR | |
MAX_SUSTAINED_WRITE_OPERATIONS_PER_MINUTE | |
Methods | |
get −
chrome.bookmarks.get(string or array of string idOrIdList, function callback)
| |
getChildren −
chrome.bookmarks.getChildren(string id, function callback)
| |
getRecent −
chrome.bookmarks.getRecent(integer numberOfItems, function callback)
| |
getTree −
chrome.bookmarks.getTree(function callback)
| |
getSubTree −
chrome.bookmarks.getSubTree(string id, function callback)
| |
search −
chrome.bookmarks.search(string or object query, function callback)
| |
create −
chrome.bookmarks.create(object bookmark, function callback)
| |
move −
chrome.bookmarks.move(string id, object destination, function callback)
| |
update −
chrome.bookmarks.update(string id, object changes, function callback)
| |
remove −
chrome.bookmarks.remove(string id, function callback)
| |
removeTree −
chrome.bookmarks.removeTree(string id, function callback)
| |
Events | |
onCreated | |
onRemoved | |
onChanged | |
onMoved | |
onChildrenReordered | |
onImportBegan | |
onImportEnded |
Types
BookmarkTreeNodeUnmodifiable
Enum |
---|
"managed"
|
BookmarkTreeNode
properties | ||
---|---|---|
string | id |
The unique identifier for the node. IDs are unique within the current profile, and they remain valid even after the browser is restarted. |
string | (optional) parentId |
The |
integer | (optional) index |
The 0-based position of this node within its parent folder. |
string | (optional) url |
The URL navigated to when a user clicks the bookmark. Omitted for folders. |
string | title |
The text displayed for the node. |
double | (optional) dateAdded |
When this node was created, in milliseconds since the epoch ( |
double | (optional) dateGroupModified |
When the contents of this folder last changed, in milliseconds since the epoch. |
BookmarkTreeNodeUnmodifiable | (optional) unmodifiable |
Since Chrome 37. Indicates the reason why this node is unmodifiable. The managed value indicates that this node was configured by the system administrator or by the custodian of a supervised user. Omitted if the node can be modified by the user and the extension (default). |
array of BookmarkTreeNode | (optional) children |
An ordered list of children of this node. |
Properties
1,000,000 |
chrome.bookmarks.MAX_WRITE_OPERATIONS_PER_HOUR |
Deprecated since Chrome 38. Bookmark write operations are no longer limited by Chrome. |
1,000,000 |
chrome.bookmarks.MAX_SUSTAINED_WRITE_OPERATIONS_PER_MINUTE |
Deprecated since Chrome 38. Bookmark write operations are no longer limited by Chrome. |
Methods
get
chrome.bookmarks.get(string or array of string idOrIdList, function callback)
Retrieves the specified BookmarkTreeNode(s).
Parameters | |||||
---|---|---|---|---|---|
string or array of string | idOrIdList |
A single string-valued id, or an array of string-valued ids |
|||
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
getChildren
chrome.bookmarks.getChildren(string id, function callback)
Retrieves the children of the specified BookmarkTreeNode id.
Parameters | |||||
---|---|---|---|---|---|
string | id | ||||
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
getRecent
chrome.bookmarks.getRecent(integer numberOfItems, function callback)
Retrieves the recently added bookmarks.
Parameters | |||||
---|---|---|---|---|---|
integer | numberOfItems |
The maximum number of items to return. |
|||
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
getTree
chrome.bookmarks.getTree(function callback)
Retrieves the entire Bookmarks hierarchy.
Parameters | |||||
---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
getSubTree
chrome.bookmarks.getSubTree(string id, function callback)
Retrieves part of the Bookmarks hierarchy, starting at the specified node.
Parameters | |||||
---|---|---|---|---|---|
string | id |
The ID of the root of the subtree to retrieve. |
|||
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
search
chrome.bookmarks.search(string or object query, function callback)
Searches for BookmarkTreeNodes matching the given query. Queries specified with an object produce BookmarkTreeNodes matching all specified properties.
Parameters | |||||
---|---|---|---|---|---|
string or object | query |
Either a string of words and quoted phrases that are matched against bookmark URLs and titles, or an object. If an object, the properties |
|||
function | callback |
The callback parameter should be a function that looks like this: function(array of BookmarkTreeNode results) {...};
|
create
chrome.bookmarks.create(object bookmark, function callback)
Creates a bookmark or folder under the specified parentId. If url is NULL or missing, it will be a folder.
Parameters | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
object | bookmark |
|
||||||||||||
function | (optional) callback |
If you specify the callback parameter, it should be a function that looks like this: function( BookmarkTreeNode result) {...};
|
move
chrome.bookmarks.move(string id, object destination, function callback)
Moves the specified BookmarkTreeNode to the provided location.
Parameters | ||||||||
---|---|---|---|---|---|---|---|---|
string | id | |||||||
object | destination |
|
||||||
function | (optional) callback |
If you specify the callback parameter, it should be a function that looks like this: function( BookmarkTreeNode result) {...};
|
update
chrome.bookmarks.update(string id, object changes, function callback)
Updates the properties of a bookmark or folder. Specify only the properties that you want to change; unspecified properties will be left unchanged. Note: Currently, only 'title' and 'url' are supported.
Parameters | ||||||||
---|---|---|---|---|---|---|---|---|
string | id | |||||||
object | changes |
|
||||||
function | (optional) callback |
If you specify the callback parameter, it should be a function that looks like this: function( BookmarkTreeNode result) {...};
|
remove
chrome.bookmarks.remove(string id, function callback)
Removes a bookmark or an empty bookmark folder.
Parameters | ||
---|---|---|
string | id | |
function | (optional) callback |
If you specify the callback parameter, it should be a function that looks like this: function() {...};
|
removeTree
chrome.bookmarks.removeTree(string id, function callback)
Recursively removes a bookmark folder.
Parameters | ||
---|---|---|
string | id | |
function | (optional) callback |
If you specify the callback parameter, it should be a function that looks like this: function() {...};
|
Events
onCreated
Fired when a bookmark or folder is created.
addListener
chrome.bookmarks.onCreated.addListener(function callback)
Parameters | ||||||||
---|---|---|---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(string id, BookmarkTreeNode bookmark) {...};
|
onRemoved
Fired when a bookmark or folder is removed. When a folder is removed recursively, a single notification is fired for the folder, and none for its contents.
addListener
chrome.bookmarks.onRemoved.addListener(function callback)
Parameters | |||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(string id, object removeInfo) {...};
|
onChanged
Fired when a bookmark or folder changes. Note: Currently, only title and url changes trigger this.
addListener
chrome.bookmarks.onChanged.addListener(function callback)
Parameters | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(string id, object changeInfo) {...};
|
onMoved
Fired when a bookmark or folder is moved to a different parent folder.
addListener
chrome.bookmarks.onMoved.addListener(function callback)
Parameters | ||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(string id, object moveInfo) {...};
|
onChildrenReordered
Fired when the children of a folder have changed their order due to the order being sorted in the UI. This is not called as a result of a move().
addListener
chrome.bookmarks.onChildrenReordered.addListener(function callback)
Parameters | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function(string id, object reorderInfo) {...};
|
onImportBegan
Fired when a bookmark import session is begun. Expensive observers should ignore onCreated updates until onImportEnded is fired. Observers should still handle other notifications immediately.
addListener
chrome.bookmarks.onImportBegan.addListener(function callback)
Parameters | ||
---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function() {...};
|
onImportEnded
Fired when a bookmark import session is ended.
addListener
chrome.bookmarks.onImportEnded.addListener(function callback)
Parameters | ||
---|---|---|
function | callback |
The callback parameter should be a function that looks like this: function() {...};
|